To dump/download an wsdl file including all its imported wsdl and xsd files, you can use the svcutil tool available in the Microsoft SDK.
How to use:
- 1. open a command prompt
- 2. change directory to the windows SDK bin directory: cd "C:\Program Files\Microsoft SDKs\Windows\v7.0A\bin"
- 3. type the following command in the prompt: svcutil /t:metadata /directory:D:\some-directory http://your-wsdl-url.wsdl
The result is all the files will be dumped to the D:\some-directory folder, all the unnecessary things (like comments) will be skipped, and you will get a clean wsdl.
Example dumping ONVIF deviceio.wsdl from onvif.org: svcutil /t:metadata /directory:D:\onvif http://www.onvif.org/onvif/ver10/deviceio.wsdl
The result:
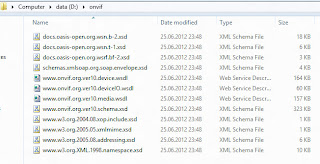
Have a nice day.